· Techtribe team · Tutorials · 3 min read
Mastering Pointers in Go: A Practical Guide
Learn how pointers work in Go, from memory management to dereferencing, with simple analogies.
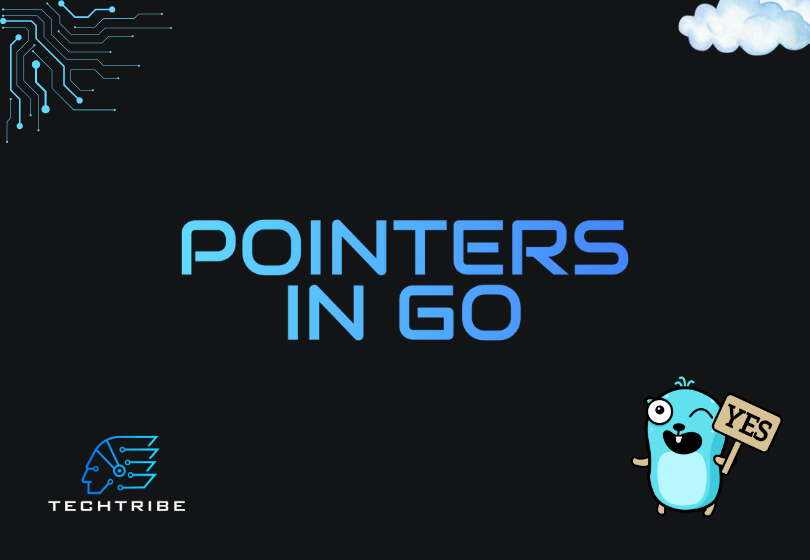
Table of Contents
Hello, and welcome! Today, we’re going to demystify pointers in Go by using a simple office analogy
About Variables
But before talking about pointers, first we have to think about variables. When we think about a variable in go, we would have a name, type and value.
Once our code is running, that variable will be stored somewhere in memory
Now, imagine we’re in a busy office and that office have a lot of cubicles. Each of these cubicles are workstations and have an employee working inside.
Let’s say we have an employee named John who is an accountant. John is working in one of these cubicles, but to find John we don’t just need to know his name or job title. We need to know which workstation he’s in. The workstation could be our variable, for sake of simplicity, lets name this workstation “John” and since John is such a great employee, let’s give him the value 100.
But to correctly manage our office, the variable should also have an address
About pointers
The address is very important, because it will allow us to easily find our variable. So when we talk about pointers, we can think of them as variables that store the memory address of another variable.
In Go, when we declare a variable, it’s like assigning an employee to a cubicle. Let’s see an example.
First we are gonna assign John the value of 100
var john int = 100
As John have an address that he’s in, we can store it in another variable
var ptr *int = &john
Now, lets print both this variables in order:
func main() {
var john int = 100
var ptr *int = &john
println(john)
println(ptr)
}
Think for a second and think, what will that print?
As you can see, the first print gave us John’s variable value, and the second one gave us the address of John’s variable in memory.
So, in order to create a pointer, we use an asterisk (*) before the type.
And the ampersand (&) to get the address of our variable, so the variable ptr will be the card that holds the address of John
We could’ve simply printed the address of john’s variable directly using the ampersand syntax:
So everytime you read a go code and see an ampersand you can really think of it as being an “address of”
Dereferencing
What If we print *ptr what might happen? Pause the video and think for a minute
We will get the value inside john’s variable. So everytime we use the asterisk (*) operator, we will get the value of the variable that the pointer is pointing to, and not the address
We can even use the value of ptr on a new variable, without modifying the value of john
Holding a pointer on another
We can even hold a pointer on another one.
When we do that, we still use the asterisk syntax, but we have to add one more asterisk to do so.
And we don’t need to stop here! We can keep going as needed
Let’s recap everything that we have learned today!