· Techtribe team · Tutorials · 5 min read
Mastering Bitwise Operators in Go: Network Programming Examples - Part 1
Learn how bitwise operators work with Network programming in Go
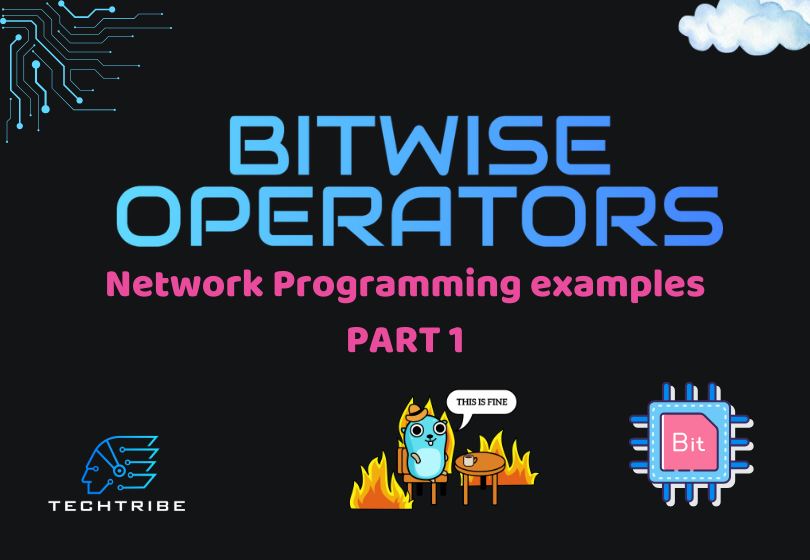
If you want to see the video, feel free to do so: https://youtu.be/Eid48qBIFrs?si=Gc1dheyaZhOZ_0-n
Hello and welcome to TechTribe! I’m Victor, your host for today. As a backend and embedded systems developer, I’m excited to take you on a deep dive into bitwise operators. In this video, we’ll explore how they work and see their practical applications in Golang network programming. Let’s get started!
Bitwise Operators might seem like a thing of the past, from an age when computers had very limited memory. Although they were used more frequently in the past, they remain highly relevant today.
These operators work directly on the individual bits of a number, making them powerful tools when you are working close to the hardware or dealing with fields where performance is critical.
You will often encounter them in scenarios such as:
- Security and encryption algorithms
- Compression techniques
- Network protocols and algorithms
- Embedded systems
One thing to remember is that 1 byte occupies 8 bits. One of the main reasons is because back in the days, we needed a way to uniform each piece of information into characters and other symbols. The ASCII table (or American Standard Code for Information Interchange) was the most popular option used as a standard to translate bits into other types of information. All the information needed by the ASCII table could fit in 8 bits, and that was one of the main reasons why 1 byte is the minimum addressable memory space in most systems still.
A common example about this fact, is that a boolean uses only 1 bit, because it can be either 1 or 0, but inside a boolean variable it takes a whole 1 byte (or 8 bits) from memory, so 7 bits are wasted memory. With that knowledge and depending on the software, optimizing that could be crucial for performance.
Knowing about the amount of bits a byte occupies is key to work with this type of operator. As we dive into bitwise operations, you will see how Go makes use of these operators, especially when working with networking tasks that rely on manipulating bits to handle protocols, masks and addresses.
In the following table, we have lísted all the bitwise operators in Go, and how they work. You don’t need to hustle to understand all of them now, because we will cover each of them step by step along the way.
Golang Network example - AND & - Subnet Mask
One great way of thinking about this operators is to imagine how Light Switches work when lighting Rooms. Let’s imagine a hallway that connects rooms in a house, and each room has a light switch. The state of each light switch can either be ON or OFF. Imagine bitwise operators as rules that you use to control multiple light switches (or bits) at once.
In our Go example we will use the and operator to perform subnet masking, an important operation on network programming, but before that lets talk about key networking concepts we will use in our example.
The and bitwise operator, if either switches are OFF, the light on the hallway will be OFF, because the AND operator will only be true, if both of the operands are true.
When we are talking about networking the idea is to simply have at least two devices communicating.
The internet is the biggest public network of our planet and it consists of various smaller groups and divisions of networks called subnets.
Subnets exists in various forms, they could be one network inside of an organization, so an organization could have a separate subnet for each department, and even your home network.
The Internet Protocol is another key concept, it’s a set of guidelines that tells devices how to identify themselves and communicate with each other on a network. A key part of IP is the IP address, which is used to recognize each device
The internet protocol uses the concepts of IP address. An IP address is an identifier given to each device on a network so data knows where to go. It has four parts separated by dots, with each part being a number between 0 and 255. These parts are called octets, and when put together, they make up 32 bits. Let’s see how an IPv4 address looks like
The IP address carries two infos: the network address and the host address.
The subnet mask is a 32 bitmask number that splits the IP Address into network and host. It’s a 32 bit number just like IP address
By using the Subnet Mask and performing an AND operation on the IP address to easily get the network address.
But why performing a bitwise AND operation with Subnet mask and the IP Address will give us the network address?
For the AND operator, we will check which are the common values.
Now that we understood how this process will work out, let’s see a practical example on go code, let’s get the network address using only the IP Address and the subnet mask.
And as simple as that, just by performing AND operations we got the network address of our network, pretty cool right?
Golang Network example - OR | - Broadcast Address
Now that you understand how the & operator works in network programming in Go, let’s cover some key real life examples on how you could use the or bitwise operator.
Before going to our coding example, let’s what is a broadcast in networking.
Each network or subnet has a special broadcast address that allows all devices on the network to receive a message. Broadcasts let you send information or services to every device on the network without needing to know each one’s IP address. For example, routers in a local network use the broadcast address to send HELLO messages to all devices, switches, and other routers. This helps them stay connected, maintain communication, and discover other devices close to them on the network.